A reader recently commented:
"Have you written a book on Ruby + Windows? I'd love to see the material expanded and put down on paper. I suppose I can print it out myself, but it's just not the same."
I've been asked about this before. While I currently have no such "Ruby on Windows" book to offer, I can now say that I have finally started on it.
I welcome any comments, suggestions, or advice you may have -- as readers and/or writers.
Monday, June 30, 2008
Ruby on Windows: The Book
Posted by
David Mullet
at
7:19 AM
12
comments
Sunday, June 22, 2008
Automating Excel: Creating Charts
They say a picture is worth a thousand words, and sometimes a chart can make your point more effectively than the raw data behind it can. This is especially true when comparing relative values such as monthly revenue data or team statistics.
In our last episode, we looked at how to define titles in existing Excel charts. Sticking with the Excel Charts theme, let's now investigate how to create new charts in Excel.
A chart is a visualization of data, and for this example, the data represents Runs Scored and Runs Allowed for American League Baseball teams, as reported on the mlb.com website. Our Excel worksheet contains a row for each team, with columns for Runs Scored and Runs Allowed:
We'll use the win32ole library for automating Excel, and we'll connect to a running instance of Excel and use the already open 'mlb_stats.xls' workbook:
require 'win32ole'
xl = WIN32OLE.connect('Excel.Application')
wb = xl.Workbooks('mlb_stats.xls')
Let's define some parameter variables that we'll use later:
xlColumns = 2
xlColumnClustered = 51
xlWhite = 2
xlRed = 3
xlBlue = 5
xlGray = 15
To add a new Chart object to the workbook's Charts collection, call the Charts.Add method:
mychart = wb.Charts.Add
mychart.Name = "MLB Scoring"
The Charts.Add method returns a reference to the newly-created Chart object, which we've assigned to the variable mychart.
To delete an existing Chart object, call the Charts(chart).Delete method, where chart is the name or (1-based) index of the chart to delete:
wb.Charts("MLB Scoring").Delete
wb.Charts(1).Delete
Naturally, we can't produce a chart without data, and we use the SetSourceData method to define the source of the data that the chart will represent. This method takes 2 arguments, [1] a range of cells from a worksheet, and [2] a number that indicates whether to plot the graph by rows (1) or by columns (2):
mychart.SetSourceData wb.Worksheets("Runs Scored and Allowed").Range("A1:C15"), xlColumns
There are many different types of charts to choose from, and for our purpose, we'll use a 2-dimensional column [aka vertical bars] chart, setting the ChartType property to 51 (via our previously-defined xlColumnClustered variable):
mychart.ChartType = xlColumnClustered
Now, you could execute your code at this point and create a new chart. But let's tweak the colors a bit. The SeriesCollection object holds each series of data and its related properties. Our chart has 2 series, one for Runs Scored, and one for Runs Allowed. Let's make the Runs Scored columns blue and the Runs Allowed columns red, via the Interior.ColorIndex property:
mychart.SeriesCollection(1).Interior.ColorIndex = xlBlue
mychart.SeriesCollection(2).Interior.ColorIndex = xlRed
We could also dictate the name that is displayed for each series in the legend:
mychart.SeriesCollection(1).Name = "Runs Scored"
mychart.SeriesCollection(2).Name = "Runs Allowed "
If you do not define a name for each series, Excel will try to pull it from your source data worksheet.
The PlotArea represents the area on which the data (columns, in our case) is plotted. The ChartArea is the area surrounding the PlotArea, on which the title, axes, and legend are placed. For demonstration purposes, let's define specific colors for these objects by setting their Interior.ColorIndex property:
mychart.ChartArea.Interior.ColorIndex = xlWhite
mychart.ChartArea.Border.ColorIndex = xlBlue
mychart.PlotArea.Interior.ColorIndex = xlGray
mychart.PlotArea.Border.ColorIndex = xlWhite
Finally, we'll add a title to the top of the chart, and format the text:
mychart.HasTitle = true
mychart.ChartTitle.Characters.Text = "American League - Runs Scored vs. Runs Allowed"
mychart.ChartTitle.Font.Name = 'Verdana'
mychart.ChartTitle.Font.Size = 16
mychart.ChartTitle.Font.Bold = true
Note that we first have to set the HasTitle property to true. Without first doing this, trying to define the various ChartTitle properties will raise an error.
Our complete code looks like this, and produces a chart that looks like this:
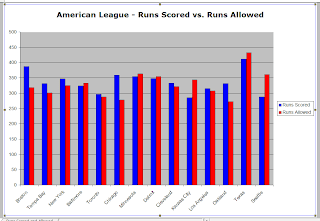
Not a bad start, eh? A quick glance at this chart tells you that Boston and Chicago are doing very well, run-wise, and that it could be a very long season for Kansas City and Seattle. And we see that Texas scores a tremendous amount of runs, but allows even more.
I encourage you to investigate the vast array of chart methods and properties. Towards that end, you might want to check out Jon Peltier's Chart tutorials, which I have found to be helpful.
Questions? Comments? Suggestions? Post a comment here or send me an email.
Thanks for stopping by!
Posted by
David Mullet
at
8:00 AM
12
comments
Sunday, June 15, 2008
Automating Excel: Chart, Axis, and Legend Titles
A reader writes, "I need to use Ruby to automate Excel... How can I set the chart title, axes title, or legend name, etc in the chart by using Ruby?" I confess that my knowledge of Excel charts is limited, but let's dive in and see what we can learn...
As usual, you'll start by using the win32ole library. For our example, we'll connect to a running instance of Excel:
require 'win32ole'
xl = WIN32OLE.connect('Excel.Application')
Referencing a Chart Object
Let's assume that ws is your worksheet object. You can obtain a reference to a Chart object either...
...via the Charts collection, if it is a chart sheet...
chart = ws.Charts(1).Chart
or via the ChartObjects collection, if it is an embedded chart (not a chart sheet)...
chart = ws.ChartObjects(1).Chart
...or via the ActiveChart method if the chart is the currently active object:
chart = xl.ActiveChart
Setting the Chart Title
In order to set the value of a chart's title, you must ensure that the HasTitle property is enabled:
chart.HasTitle = true
Then set the value of the ChartTitle.Text property:
chart.ChartTitle.Text = "2008 Sales"
Setting the Axes Title
Select one of the chart's axes by calling the Chart object's Axes method and passing it an integer representing either the category, value, or series axis:
XL_CATEGORY = 1
XL_VALUE = 2
XL_SERIESAXIS = 3
axis = chart.Axes(XL_CATEGORY)
Much like with the chart title above, in order to set the value of an axis' title, you must ensure that the HasTitle property is enabled:
axis.HasTitle = true
Then set the value of the ChartTitle.Text property:
axis.AxisTitle.Text = "July Sales"
Setting the Legend Names
You can reference a legend via the Chart object's SeriesCollection method, passing it the (1-based) index of the item you wish to reference:
chart.SeriesCollection(1).Name = "Jan"
chart.SeriesCollection(2).Name = "Feb"
chart.SeriesCollection(3).Name = "Mar"
And there you have it. If you found this to be useful, you may want to check out this Microsoft MSDN Chart object reference.
Got a question, comment, or suggestion? Post your comment here or send me an email.
Thanks for stopping by!
Posted by
David Mullet
at
3:00 AM
8
comments
Tuesday, June 3, 2008
Running In A Console Window?
Someone recently asked me how to determine if your code is running in a console window or not. Perhaps you have a program that provides both GUI and command line interfaces and you need to know which interface is being used. You may wish to output error messages to the console, if it exists, but to a file if the console doesn't exist.
UPDATE: Perhaps the preferred means for this is the STDIN.isatty method, which returns true if running in a console window and false otherwise...
if STDIN.isatty
# do some command-line stuff
else
# do some GUI stuff
end
Thanks to Brent and Dan for their comments.
Alternatively, Nobu Nakada mentioned in a recent Ruby Forum post that you cannot open the CONIN$ (console input) device file unless you are running in a console window. So the following method (adapted from Nobu) would return true if running in a console window and false otherwise...
def in_console?
begin
open("CONIN$") {}
console = true
rescue SystemCallError
console = false
end
return console
end
Usage example:
if in_console?
# do some command-line stuff
else
# do some GUI stuff
end
There you have it. Got a question or suggestion? Post a comment or send me email.
Thanks for stopping by!
Posted by
David Mullet
at
7:23 PM
3
comments
Labels: ruby